C programming code
If you want you can copy blank into text string so that original string is modified.
Download Remove spaces program.
Output of program:
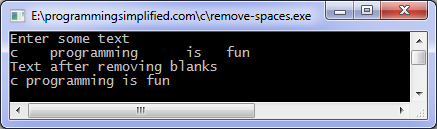
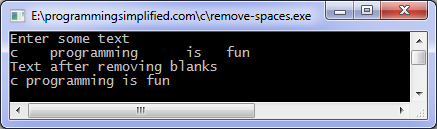
#include <stdio.h> int main() { char text[100], blank[100]; int c = 0, d = 0; printf("Enter some text\n"); gets(text); while (text[c] != '\0') { if (!(text[c] == ' ' && text[c+1] == ' ')) { blank[d] = text[c]; d++; } c++; } blank[d] = '\0'; printf("Text after removing blanks\n%s\n", blank); return 0; }
#include <stdio.h> #include <string.h> #include <stdlib.h> #define SPACE ' ' int main() { char string[100], *blank, *start; int length, c = 0, d = 0; printf("Enter a string\n"); gets(string); length = strlen(string); blank = string; start = (char*)malloc(length+1); if ( start == NULL ) exit(EXIT_FAILURE); while(*(blank+c)) { if ( *(blank+c) == SPACE && *(blank+c+1) == SPACE ) {} else { *(start+d) = *(blank+c); d++; } c++; } *(start+d) = '\0'; printf("%s\n", start); free(start); return 0; }
No comments:
Post a Comment