C program to find string length
#include <stdio.h>
#include <string.h>
int main()
{
char a[100];
int length;
printf("Enter a string to calculate it's length\n");
gets(a);
length = strlen(a);
printf("Length of entered string is = %d\n",length);
return 0;
}
Output of program:
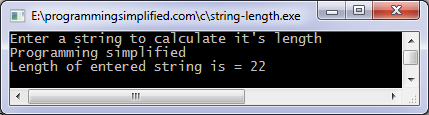
You can also find string length using pointer or without strlen function. Following program shows how to achieve this.
C program to find string length without strlen
C program to find length of a string using pointers.
#include <stdio.h>
int main()
{
char array[100], *pointer;
int length = 0;
printf("Enter a string\n");
gets(array);
pointer = array;
while(*(pointer+length))
length++;
printf("Length of entered string = %d\n",length);
return 0;
}
Function to find string length
int string_length(char *s)
{
int c = 0;
while(*(s+c))
c++;
return c;
}
No comments:
Post a Comment