C programming code
Explanation of "count[string[c]-'a']++", suppose input string begins with 'a' so c is 0 initially and string[0] = 'a' and string[0]-'a' = 0 and we increment count[0] i.e. a has occurred one time and repeat this till complete string is scanned.
Download Character frequency program.
Output of program:
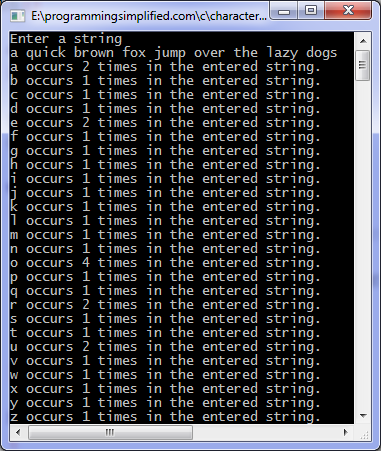
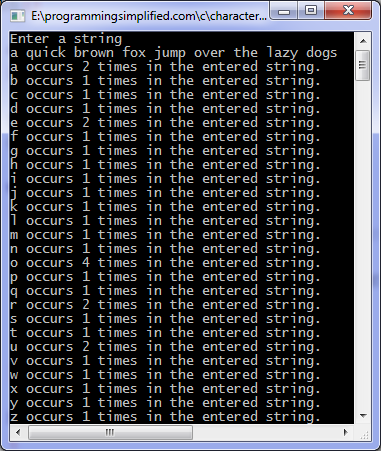
Did you notice that string in the output of program contains every alphabet at least once.
No comments:
Post a Comment